Raspberry Pi | Automated script to change the password
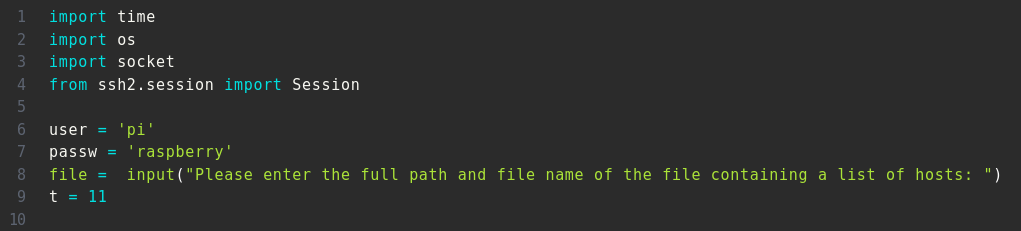
Recently I was performing a network audit and discovered a large number of Raspberry Pi devices that were configured with the default username and password (UN: pi – PW: raspberry). Since we all know that Raspberry Pi’s can be ‘low hanging fruit’ for an adversary, particularly when configured with default credentials, I decided to change the password on all of these devices.
My dilemma became, how do I change the password of 50+ raspberry pi devices without having to hunt down the owner – or have to manually log into each device and change it? That is where python enters the story. I decided to write a python script that will take a file containing a list of IP addresses as input and then go and log into each of the devices and change the password for me.
Obligatory Disclaimer: Changing the password on a remote device that you do not own is bad mojo and not recommended (and probably not legal) without written consent from either the owner or someone with the authority in an organization to take on the risk.
Here is the code that I wrote to accomplish this goal:
import time
import os
import socket
from ssh2.session import Session
user = 'pi'
passw = 'raspberry'
file = input("Please enter the full path and file name of the file containing a list of hosts: ")
t = 11
print("\n\nWARNING:\nYou are about to change the password on a remote machine. \nPlease ensure that you document the password! \nYOU H>
def countdown(t):
while t:
mins, secs = divmod(t, 60)
timeformat = '{:02d}:{:02d}'.format(mins, secs)
print(timeformat, end='\r')
time.sleep(1)
t -= 1
print('You have chosen to proceed!n\n')
countdown(int(t))
with open(file) as f:
hosts = f.readlines()
def chgpw():
channel = session.open_session()
channel.shell()
channel.write("echo raspberry | sudo -S su && echo 'pi:newP@ssw0rdHere' | sudo chpasswd\n")
time.sleep(1)
channel.close()
print ("Exit status: {0}".format(channel.get_exit_status()))
for i in hosts:
try:
stripped = i.rstrip()
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(5)
sock.connect((stripped, 22))
session = Session()
session.handshake(sock)
session.userauth_password(user, passw)
chgpw()
except:
pass
As you can see, the code is fairly simple and only requires that you install the ssh2-python module to run it. There is room for improvement, such as adding some error handling so that you can capture any devices that failed to have the password changed. I tested it on 5 machines initially, and it did the job, and can be adapted to any Linux machine, not just Raspberry Pi.
Happy Hacking!